Plotting and visualisation of inference results
This module provides functions to generate common types of plots used to visualise inference results.
matrix_plot
- inference.plotting.matrix_plot(samples, labels=None, show=True, reference=None, filename=None, plot_style='contour', colormap='Blues', show_ticks=None, point_colors=None, hdi_fractions=(0.35, 0.65, 0.95), point_size=1, label_size=10)
Construct a ‘matrix plot’ for a set of variables which shows all possible 1D and 2D marginal distributions.
- Parameters
samples – A list of array-like objects containing the samples for each variable.
labels – A list of strings to be used as axis labels for each parameter being plotted.
show (bool) – Sets whether the plot is displayed.
reference – A list of reference values for each parameter which will be over-plotted.
filename (str) – File path to which the matrix plot will be saved (if specified).
plot_style (str) – Specifies the type of plot used to display the 2D marginal distributions. Available styles are ‘contour’ for filled contour plotting, ‘hdi’ for highest-density interval contouring, ‘histogram’ for hexagonal-bin histogram, and ‘scatter’ for scatterplot.
show_ticks (bool) – By default, axis ticks are only shown when plotting less than 6 variables. This behaviour can be overridden for any number of parameters by setting show_ticks to either True or False.
point_colors – An array containing data which will be used to set the colors of the points if the plot_style argument is set to ‘scatter’.
point_size – An array containing data which will be used to set the size of the points if the plot_style argument is set to ‘scatter’.
hdi_fractions – The highest-density intervals used for contouring, specified in terms of the fraction of the total probability contained in each interval. Should be given as an iterable of floats, each in the range [0, 1].
label_size (int) – The font-size used for axis labels.
Create a spatial axis and use it to define a Gaussian process
from numpy import linspace, zeros, subtract, exp
N = 8
x = linspace(1, N, N)
mean = zeros(N)
covariance = exp(-0.1 * subtract.outer(x, x)**2)
Sample from the Gaussian process
from numpy.random import multivariate_normal
samples = multivariate_normal(mean, covariance, size=20000)
samples = [samples[:, i] for i in range(N)]
Use matrix_plot
to visualise the sample data
from inference.plotting import matrix_plot
matrix_plot(samples)
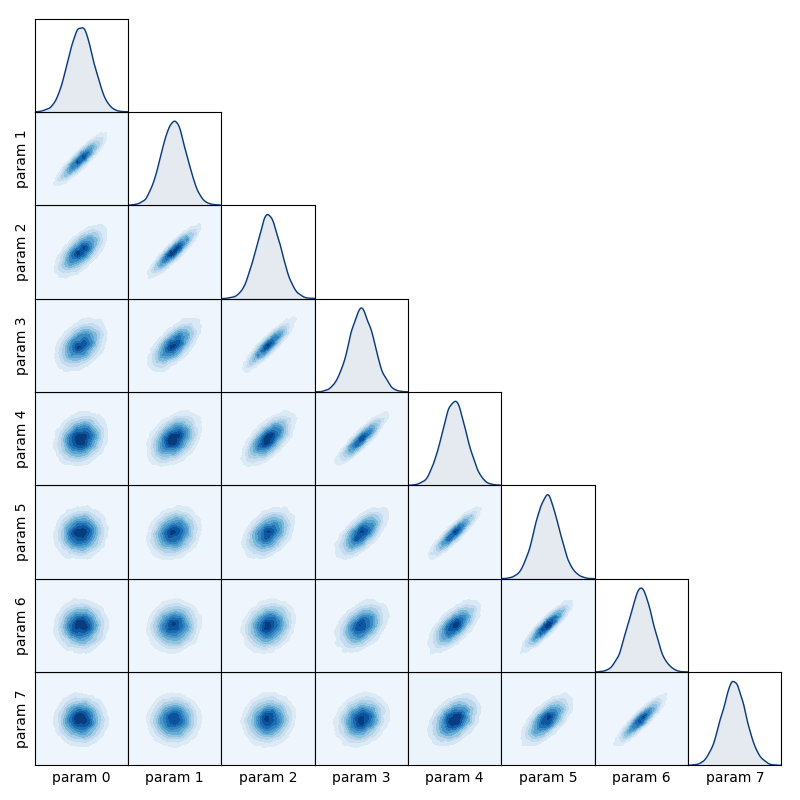
trace_plot
- inference.plotting.trace_plot(samples, labels=None, show=True, filename=None)
Construct a ‘trace plot’ for a set of variables which displays the value of the variables as a function of step number in the chain.
- Parameters
samples – A list of array-like objects containing the samples for each variable.
labels – A list of strings to be used as axis labels for each parameter being plotted.
show (bool) – Sets whether the plot is displayed.
filename (str) – File path to which the matrix plot will be saved (if specified).
hdi_plot
- inference.plotting.hdi_plot(x, sample, intervals=(0.65, 0.95), colormap='Blues', axis=None, label_intervals=True, color_levels=None)
Plot highest-density intervals for a given sample of model realisations.
- Parameters
x – The x-axis locations of the sample data.
sample – A
numpy.ndarray
containing the sample data, which has shape(n, len(x))
wheren
is the number of samples.intervals – A tuple containing the fractions of the total probability for each interval.
colormap – The colormap to be used for plotting the intervals. Must be a vaild argument of the
matplotlib.cm.get_cmap
function.axis – A
matplotlib.pyplot
axis object which will be used to plot the intervals.label_intervals (bool) – If
True
, then labels will be assigned to each interval plot such that they appear in the legend when usingmatplotlib.pyplot.legend
.color_levels – A list of integers in the range [0,255] which specify the color value within the chosen color map to be used for each of the intervals.